The Kvaser C# wrapper for CANlib has been ported to .NET Standard. In this document, I will show you how to enable CANlib in Visual Studio 2019 when creating a C# project using the .NET Standard 2.0 for both “.NET CORE” and “.NET Framework”. This document can also be used when enabling CANlib in an existing project.
Developer Blog
Using CANlib Visual Studio 2019 C# .NET STANDARD 2.0
This text is based on the BLOG: Using CANlib Visual Studio 2017 C#.NET.
It has been modified for “Visual Studio 2019” and now shows how to use the “.NET Standard 2.0” framework (and later).
Abbreviations
VS2019 – Microsoft Visual Studio 2019 (C# .NET)
CANlib – Kvaser CANlib SDK
Before we start...
First we must download and install “Kvaser CANlib SDK“ and also “Kvaser Drivers for Windows”. After you have installed CANlib, please check where Kvaser CANlib “netstandard2.0” has been installed. On my 64-bit Windows machine they are installed here:
- c:\Program Files (x86)\Kvaser\Canlib\dotnet\win32\netstandard2.0\
- c:\Program Files (x86)\Kvaser\Canlib\dotnet\x64\netstandard2.0\
Please remember where you found them, as you will need this information soon.
If you have the intention to create an application that supports “.NET Framework 4.6” or earlier, then you need to use “canlibCLSNET.DLL”. How to use “canlibCLSNET.DLL” is described in the blog post:Using CANlib Visual Studio 2017 C#.NET
Please also install “Microsoft Visual C++ Redistributable for Visual Studio 2015, 2017 and 2019” if you haven’t done it already. (Installs by default if you install the C++ tools when installing VS2019.) Now we are ready to start Visual Studio 2019 (C#).
Creating an empty project
Creating a project in Visual Studio can be done in many different ways; this is one way to do it.
Press, “File” – “New” -”Project…”
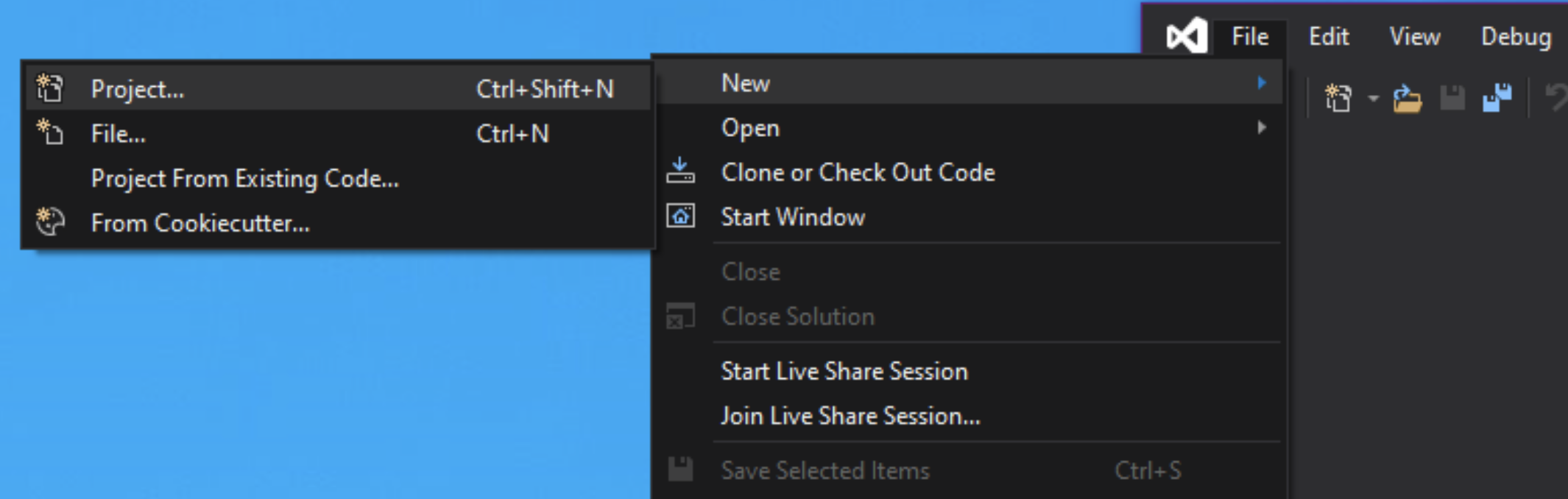
Create a “.NET Core” or “.NET Framework” project. Edit the name and the rest of the unique information and press “OK”. VS2019 now creates an application for us. It is a bit boring, just an empty form that does nothing.
Adding some code that uses CANlib (C#)
We will now add some code that uses CANlib; exactly what it does is not important in this example. For more information about how to use CANlib, please check CANlib SDK Help.
Windows Forms Designer
This information was valid 2019-11-15.
Information from Microsoft:
Because this is the very first preview of the designer, it isn’t yet bundled with Visual Studio and instead is available as a Visual Studio extension (“VSIX”) (download). That means that if you open a Windows Forms project targeting .NET Core in Visual Studio, it won’t have the designer support by default – you need to install the .NET Core Designer first!
You can find the full article here:
Introducing .NET Core Windows Forms Designer Preview 1
Editing the FORM
To add a BUTTON and a TEXTBOX, change the property “Multiline” on the textbox to true. (I will assume that you are familiar with how to add components in VS2019, so I will not show it here).
A lot of information can be found here:
After a bit of editing, we have this beautiful application.
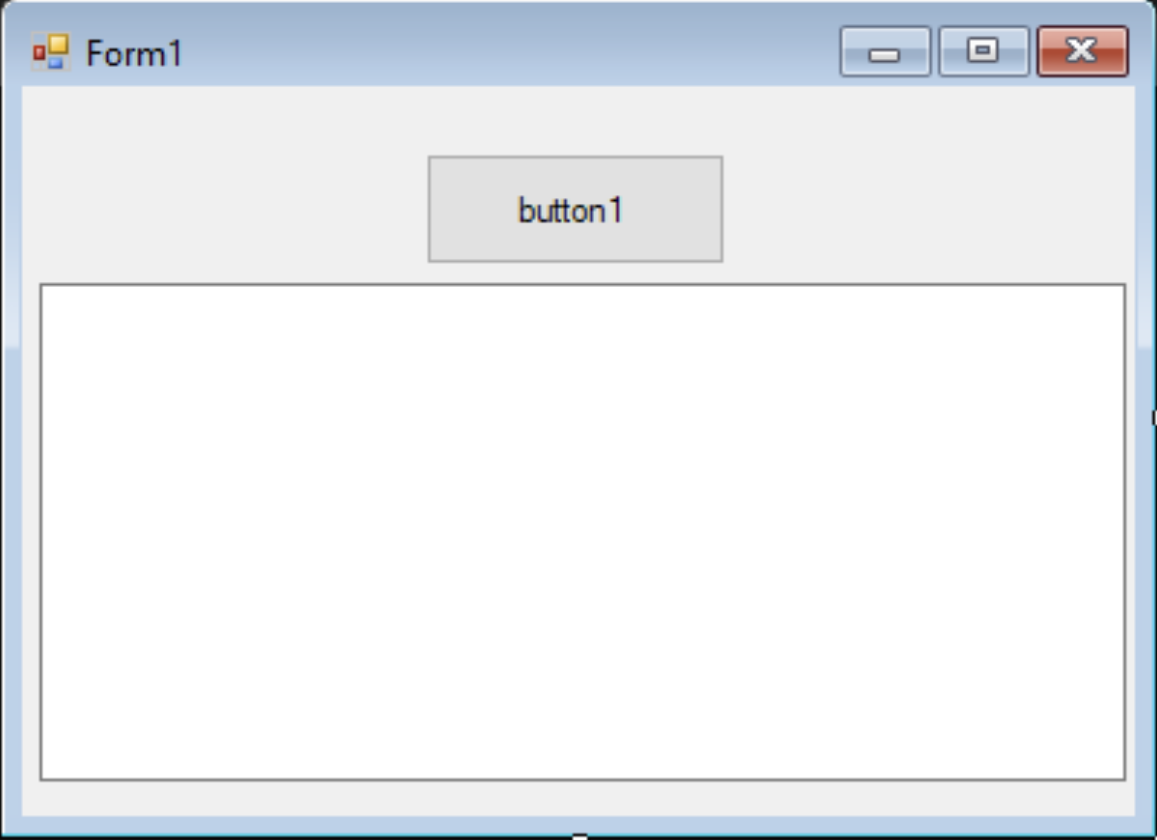
Editing the CODE
It still does nothing, so in the editor, double click on “button1” and add some code inside the “button1_Click” function.
private void button1_Click(object sender, EventArgs e)
{
Canlib.canStatus R;
int V;
textBox1.Clear();
textBox1.Text = "DEMO VS2019\r\n";
Canlib.canInitializeLibrary();
V = Canlib.canGetVersionEx(Canlib.canVERSION_CANLIB32_PRODVER32);
int V1 = (V & 0xFF0000) >> 16;
int V2 = (V & 0xFF00) >> 8;
textBox1.Text += string.Format("Found CANlib version {0}.{1}\r\n",V1,V2 );
R = Canlib.canGetNumberOfChannels(out int NOC);
textBox1.Text += string.Format("Found {0} channels\r\n", NOC);
textBox1.Text += "----------------------------------------\r\n";
}
When I try to run the application, I get multiple errors. Something is wrong as VS2019 can’t find CANlib.
Adding CANlib
In order to use CANlib in a VS2019 project, we must add information to show where VS2019 can find the CANlib files. It is only possible to add a reference to either the x86 version or the x64 version of CANlib. However, with a minor modification in one file, we can enable both. I will show that later in this document. I will add the reference to the win32 versions. My machine defaults to x64 when using “anyCPU” but it seems to work anyway.
Before we start, set the platform to “anyCPU” (for the moment, it is the only platform I have enabled by default in this application).
Adding “using Kvaser.CanLib;”
If we intend to create a “.NET Core” or “.NET Framework”(V4.6.1 or later) then we shall add using Kvaser.CanLib;
Adding Reference “Kvaser.CanLib”
In order to use CANlib in a VS2019 project, we must add information to show VS2019 where it can find Kvaser.CanLib
. In my computer I found the path earlier:
“c:\Program Files (x86)\Kvaser\Canlib\dotnet\win32\netstandard2.0\”
Right click on “References”, and select “Add Reference…” Select the wanted DLL and press OK. I selected the win32 version. (If the DLL is not visible, then select “Browse…”.)
Now we can see that the “Kvaser.CanLib” reference has been added to our list of “References.”
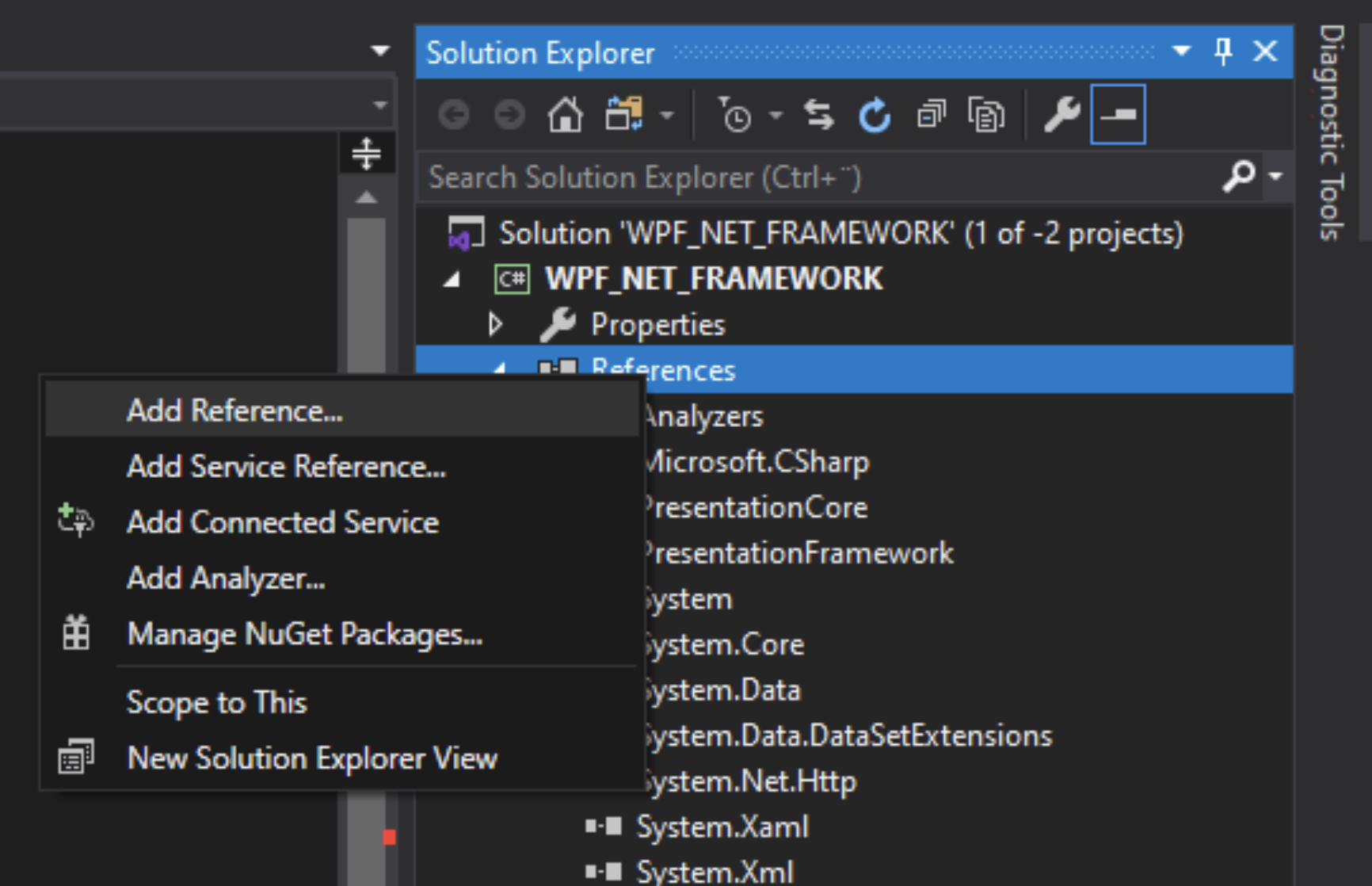
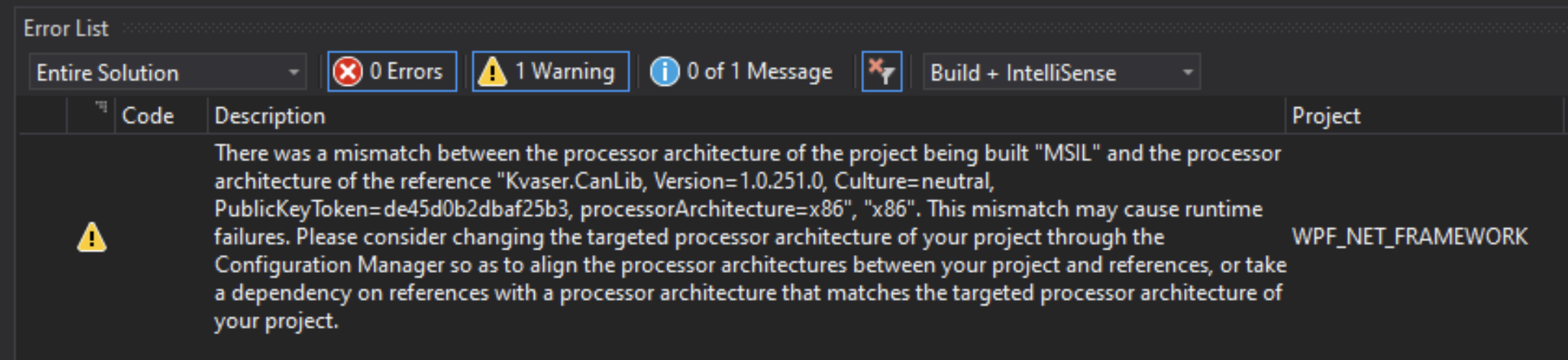
Building the application
If I try to build the application, it works! (sometimes)
This warning is normal, it will disappear when we add more information to the project.
Running an application
If I run my application and press Button1, some text will appear in the text box. The application can start CANlib, and it found some interfaces. It seems to be working.
However, sometimes I get a WARNING, and sometimes I get an ERROR. I am not sure why I get the warning or error, because I should always get the error. (I am running my application on a WIN64 PC calling a win32 DLL and the result should be an error. Maybe the compiler tries to save me.)
There is a simple fix to this little problem, and while we fix it, we also add support for the WIN32 and WIN64 application platforms. Keep on reading …
Enabling CANlib for WIN32 and WIN64
So far, we have used “anyCPU” as the target CPU platform. Now we want to set it to the platforms so we can build applications for x86 (win32) or x64 (win64).
Select “Configuration Manager…” Select “<New…>” and add both x86 and x64.
Copy settings from “anyCPU.” I do this for both x86 and x64 and then return to the main IDE.
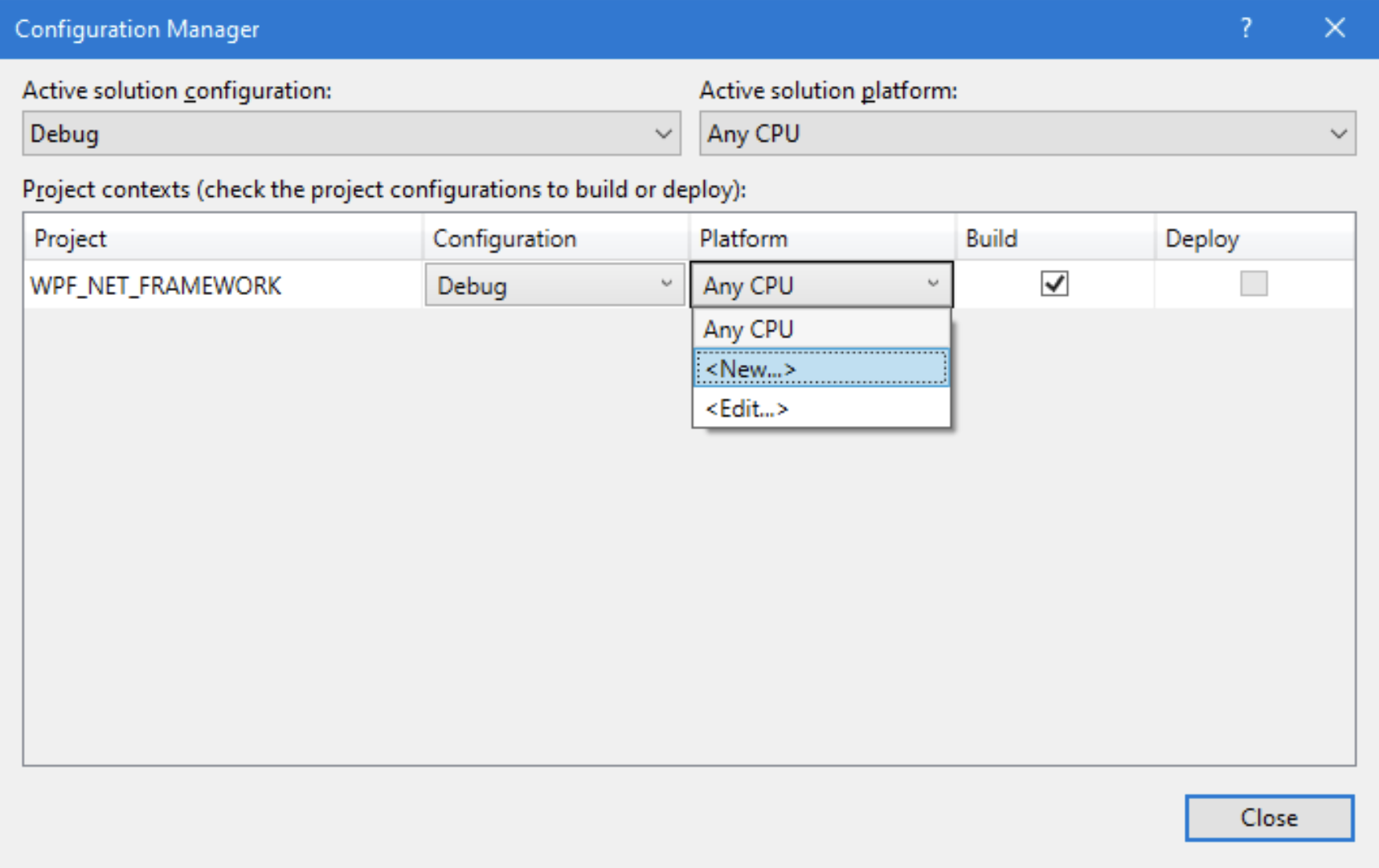
Important: Use the “new” from the “Project contexts”, not from “Active solutions platform”.
Testing x86 and x64
Select “x86” and press “Start.” It works! The application starts, but I get a warning. Select “x64” and press “Start.” I got a warning, but expected an error. This is normal and will be fixed in the next section.
Okay, now the situation has improved a lot. We can select the platform, and sometimes we get a warning, and sometimes an error. I said earlier that we would fix this, and now this seems to be a proper time to do that.
Setting PATH for Kvaser.CanLib WIN32/WIN64
I want VS2019 to automatically select the correct version of Kvaser.CanLib.dll
when I build my project. I have found one method to do it (there might be others).
The method differs depending on the project type we have created:
- Adjusting path for “.NET CORE” projects
- Adjusting path for “.NETFramework” projects
More information can be found here:
How to: Configure projects to target platforms
Adjusting path for “.NET CORE” projects
We shall now edit the file “WPF_NET_CORE.csproj” (ProjName.csproj).
Before editing, it looks like this:
<Project Sdk="Microsoft.NET.Sdk.WindowsDesktop">
<PropertyGroup> .... </PropertyGroup> (Keep original info!)
<ItemGroup>
<Reference Include="Kvaser.CanLib">
<HintPath>C:\Program Files (x86)\Kvaser\Canlib\dotnet\win32\netstandard2.0\Kvaser.CanLib.dll</HintPath>
</Reference>
</ItemGroup>
</Project>
Keep all original info, but locate the <ItemGroup>
that handles the reference to Kvaser.CanLib.dll
.
We shall now add some lines so it looks like this:
<Project Sdk="Microsoft.NET.Sdk.WindowsDesktop">
<PropertyGroup> .... </PropertyGroup> (Keep original info!)
<ItemGroup>
<Reference Include="Kvaser.CanLib">
<HintPath>C:\Program Files (x86)\Kvaser\Canlib\dotnet\win32\netstandard2.0\Kvaser.CanLib.dll</HintPath>
</Reference>
</ItemGroup>
<ItemGroup Condition=" '$(Platform)' == 'x64' ">
<Reference Include="Kvaser.CanLib">
<HintPath>C:\Program Files (x86)\Kvaser\Canlib\dotnet\x64\netstandard2.0\Kvaser.CanLib.dll</HintPath>
</Reference>
</ItemGroup>
</Project>
I added an ItemGroup with a condition (x64). This ItemGroup/Reference will override the first ItemGroup/Reference, if I have selected x64 as the platform. It is important that you add the x64 ItemGroup/Reference after the general group.
When we have done this, we are ready to continue with the next step.
Adjusting the path for “.NETFramework” projects
In my directory structure for the project, there is a file we can edit:
WPF_NET_FRAMEWORK.csproj.user (%ProjName%.csproj.user)
But I cannot find it! Where is the file? Okay, no problem. It is not created by default, only when needed. Let’s create it!
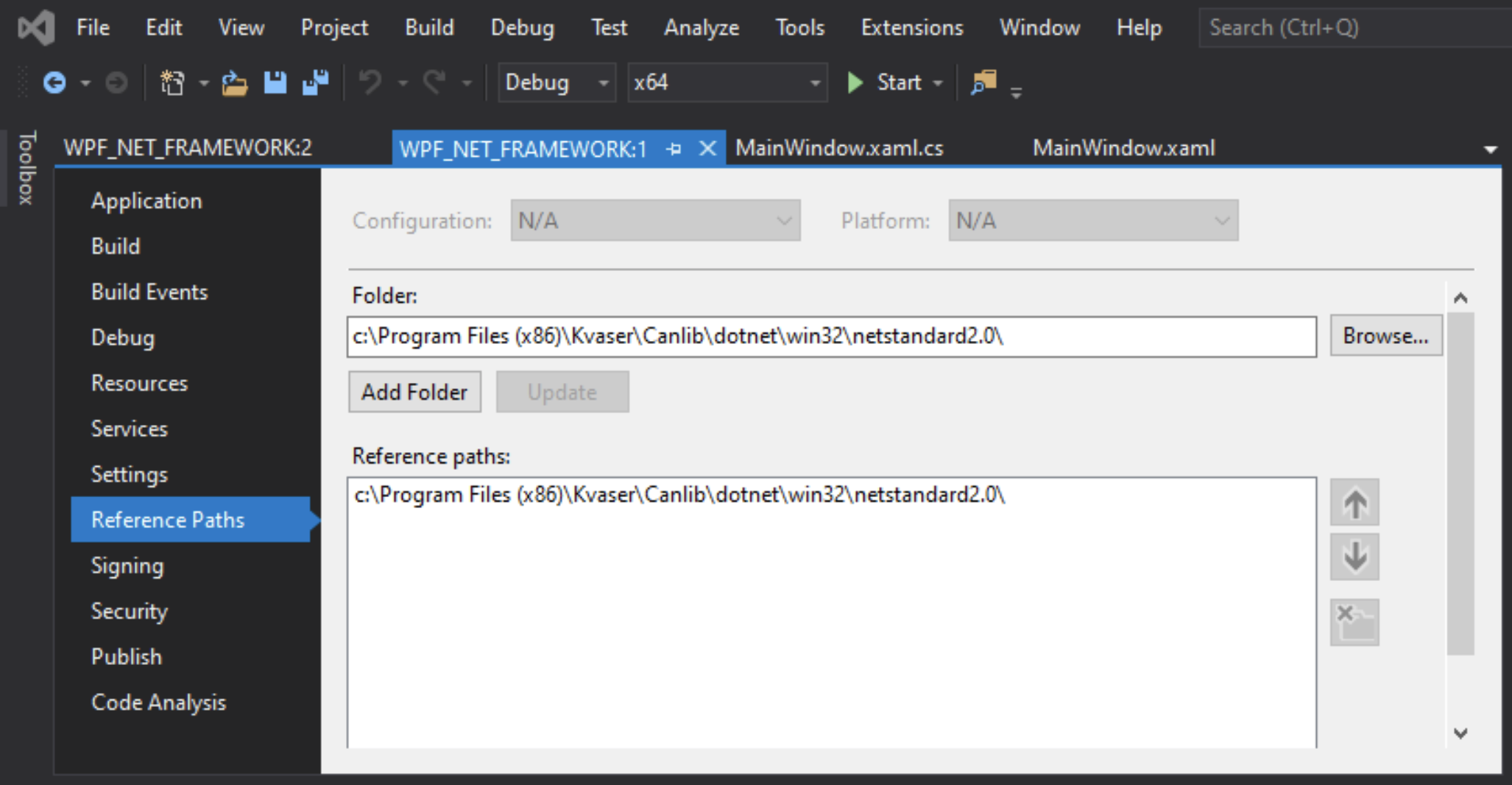
Open “Project” – %ProjName%_Properties (At the bottom), go to the section “Reference Paths” and add the path to, c:\Program Files (x86)\Kvaser\Canlib\dotnet\win32\netstandard2.0\
. Now, save the project and close VS2019. When we check our project directory, we can find the file “ProjName.csproj.user”. I open it with my text editor, and it looks like this:
(Please note, this file can contain a lot of information, so it is necessary to locate the part where the “ReferencePath” has been defined.)
?xml version="1.0" encoding="utf-8"?>
<Project ToolsVersion="Current" xmlns="http://schemas.microsoft.com/developer/msbuild/2003">
<PropertyGroup>
<ReferencePath>c:\Program Files (x86)\Kvaser\Canlib\dotnet\win32\netstandard2.0\</ReferencePath>
</PropertyGroup>
</Project>
We add property group declaration:
<PropertyGroup Condition=" '$(Platform)' == 'x64' ">
<ReferencePath>c:\Program Files (x86)\Kvaser\Canlib\dotnet\x64\netstandard2.0\</ReferencePath>
</PropertyGroup>
Now my file looks like this:
<?xml version="1.0" encoding="utf-8"?>
<Project ToolsVersion="Current" xmlns="http://schemas.microsoft.com/developer/msbuild/2003">
<PropertyGroup>
<ReferencePath>c:\Program Files (x86)\Kvaser\Canlib\dotnet\win32\netstandard2.0\</ReferencePath>
</PropertyGroup>
<PropertyGroup Condition=" '$(Platform)' == 'x64' ">
<ReferencePath>c:\Program Files (x86)\Kvaser\Canlib\dotnet\x64\netstandard2.0\</ReferencePath>
</PropertyGroup>
</Project>
I added a PropertyGroup with a condition (x64). This group will override the first PropertyGroup, if I have selected x64 as the platform. It is important that you add the x64 group after the general group.
Running on platform x86 and x64
We can now select x86 (win32) and x64 (win64) as active platforms. In both cases the application starts correctly and CANlib is available and working.
We have now enabled CANlib in Visual Studio 2019, created a C# project (for .NET Framework and Netstandard) and tested it for both x86 and x64.
ΟΕΔ (Q.E.D. Quod erat demonstrandum)
Thoughts and FAQ
Can I use anyCPU?
When I select anyCPU as a platform, I get a warning or an error!
When the computer starts Visual Studio, if I have selected anyCPU, the software will decide whether to use the 32bit or 64bit version of the DLL. Yes, anyCPU might work, but if I run the built application on another machine, with a different setup, it might not work.
(Selectable loading of the DLL might work, please check this external link for more information: https://stackoverflow.com/questions/108971/using-side-by-side-assemblies-to-load-the-x64-or-x32-version-of-a-dll)
Safest setup?
If I select x86 (WIN32) built application, it will run on both WIN32 and WIN64 machines.
Do I need x64?
If your application needs it, CANlib will work perfectly, but only on WIN64 machines. However, today (2019), the majority of Windows PCs are WIN64.
Microsoft Visual C++ and Kvaser.CanLib Redistributable
When installing your app on another machine (might not have Visual Studio installed), please remember to redistribute (and install) the “Microsoft Visual C++ Redistributable for Visual Studio 2019”. You will need to copy the Kvaser.CanLib.dll (x86 and/or x64) with your project executable. These dlls are not installed as part of the driver (they are part of KVASER CANlib SDK).
Thanks for reading this! If you have any questions or comments regarding this paper, please send a mail to support@kvaser.com.